Basics¶
The design plugin tidy3d.plugins.design
is a wrapper that makes it simple and convenient for tidy3d
users to define experiments to systematically explore a design space.
In short, users define the dimensions of their design problem, as well as the method they wish to explore the design space. These specifications are combined in a DesignSpace
object.
Then, the user passes a function that defines the input / output relationship they wish to explore. The function arguments correspond to the dimensions defined in the DesignSpace
and the function outputs can be anything.
The resulting data is stored as a Result
object, which has its own methods but can be easily converted to a pandas.DataFrame
for analysis, post processing, or visualization. The columns in this DataFrame
correspond to the function inputs and outputs and each data point corresponds to a row in this DataFrame
.
For reference, here is a diagram of the entire process.
For another example of the design
plugin used in practice, see about halfway down our parameter scan notebook. See the use of the design
plugin in a practical case study, see the all-dielectric structural color notebook.
Note: this feature is in pre-release stage. if you have questions or suggestions for the API, please feel free to make an issue on our github page.
The plugin is imported from tidy3d.plugins.design
so it's convenient to import this namespace first along with the other packages we need.
import numpy as np
import matplotlib.pylab as plt
import typing
import tidy3d as td
import tidy3d.web as web
import tidy3d.plugins.design as tdd
Quickstart¶
While the design
plugin is built on Tidy3D
and has special features for handling FDTD simulations, it can also be run with any general design problem. Here is a quick, complete example before diving into the use of the plugin with Tidy3D
simulations.
Here we want to sample a design space with two dimensions x
and y
with Monte Carlo. Our function simply returns a Gaussian centered at (0,0)
and we'll evaluate the result for Gaussians with 3 different variances.
# define your design space (parameters (x,y) within certain ranges)
param_x = tdd.ParameterFloat(name="x", span=(-15,15))
param_y = tdd.ParameterFloat(name="y", span=(-15,15))
# define your sampling method, Monte Carlo method with 10,000 points
method = tdd.MethodMonteCarlo(num_points=10000)
# put everything together in a `DesignSpace` container
design_space = tdd.DesignSpace(method=method, parameters=[param_x, param_y])
# define your figure of merit function. Here we compute a gaussian as a function of x and y with different values for the width.
sigmas = {f"sigma={s}": s for s in [5, 10, 20]}
def f(x:float, y:float) -> typing.Dict[str, float]:
"""gaussian distribution as a function of x and y."""
r2 = x**2 + y**2
gaussian = lambda sigma: np.exp(-r2 / sigma ** 2)
# return a dictionary, where the key is used to label the output names
return {key: gaussian(sigma) for key, sigma in sigmas.items()}
# note, we return a dictionary here, they keys correspond to our output data labels
# in the future, this function will involve running tidy3d simulations
# .run the design on our function and convert the results to a pandas.DataFrame
df = design_space.run(f).to_dataframe()
# plot the results using pandas.DataFrame builtins
f, axes = plt.subplots(1, 3, figsize=(10, 3), tight_layout=True)
for ax, C in zip(axes, sigmas.keys()):
_ = df.plot.hexbin(x='x', y='y', gridsize=20, C=C, cmap="magma", ax=ax)
ax.set_title(C)
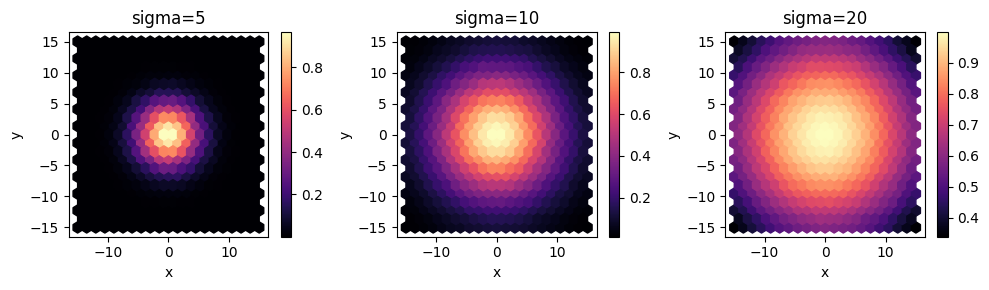
# look at the first 5 outputs
df.head()
x | y | sigma=5 | sigma=10 | sigma=20 | |
---|---|---|---|---|---|
0 | 9.703122 | -1.353683 | 0.021508 | 0.382958 | 0.786661 |
1 | 9.679707 | -0.189179 | 0.023534 | 0.391674 | 0.791100 |
2 | -13.261895 | 10.517973 | 0.000011 | 0.056980 | 0.488574 |
3 | 8.166869 | -11.831513 | 0.000257 | 0.126587 | 0.596482 |
4 | -10.947855 | 7.040571 | 0.001140 | 0.183737 | 0.654710 |
Design Space Exploration in Tidy3D¶
This is essentially the same process that we will use to perform a parameter sweep of a photonic device. The only difference is that our function evaluation each point will involve a tidy3d
simulation.
For a concrete example, let's analyze the transmission of a 1D multilayer slab. Our system will have num
layers with refractive index alternating between two values, each with thickness t
. We write a function to compute the transmitted flux through this system at frequency freq0
as a function of num
and t
.
lambda0 = 0.63
freq0 = td.C_0 / lambda0
mnt_name = "flux"
buffer = 1.5 * lambda0
refractive_indices = [1.5, 1.2]
Let's write our measurement function, for convenience, we'll break it into pre-processing and post-processing functions. The pre-processing function returns a tidy3d.Simulation
as a function of the input parameters and the post-processing function returns the transmitted flux as a function of the tidy3d.SimulationData
associated with the simulation.
def pre(num: int, t: float) -> td.Simulation:
"""Pre-processing function, which creates a tidy3d simulation given the function inputs."""
layers = []
z = 0
for i in range(num):
n = refractive_indices[i % len(refractive_indices)]
thickness = t * lambda0 / n
medium = td.Medium(permittivity = n ** 2)
geometry = td.Box(center=(0, 0, z + thickness / 2), size=(td.inf, td.inf, thickness))
layers.append(td.Structure(geometry=geometry, medium=medium))
z += thickness
Lz = z + 2 * buffer
sim = td.Simulation(
size=(0, 0, Lz),
center=(0, 0, z / 2),
structures=layers,
sources=[td.PlaneWave(
size=(td.inf, td.inf, 0),
center=(0, 0, -buffer * 0.75),
source_time=td.GaussianPulse(freq0=freq0, fwidth=freq0/10),
direction="+",
)],
monitors=[td.FluxMonitor(
size=(td.inf, td.inf, 0),
center=(0, 0, z + buffer * 0.75),
freqs=[freq0],
name=mnt_name,
)],
boundary_spec=td.BoundarySpec.pml(x=False, y=False, z=True),
run_time=100 / freq0,
)
return sim
def post(data: td.SimulationData) -> dict:
"""Post-processing function, which processes the tidy3d simulation data to return the function output."""
flux = np.sum(data['flux'].flux.values)
return {"flux": flux}
Note: if we were to write the full transmission function using pre and post it would look like the function below. However, the sweep plugin will handle running the simulation for us, as we'll see later.
def transmission(num: int, t: float) -> float:
"""Transmission as a function of number of layers and their thicknesses.
Note: not used, just a demonstration of how the pre and post functions are related.
"""
sim = pre(num=num, t=t)
data = web.run(sim, task_name=f"num={num}_t={t}")
return post(data=data)
Note: we choose to have our post-processing function return a dictionary
{"flux": flux}
instead of just afloat
. If a dictionary is returned, thedesign
plugin will use the keys to label the dataset outputs, otherwise it will use defaults, so this approach is slightly cleaner.
Let's visualize the simulation for some example parameters to make sure it looks ok.
sim = pre(num=5, t=0.4)
ax = sim.plot_eps(x=0, hlim=(-1, 1))
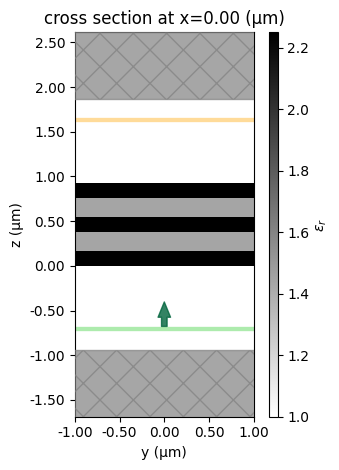
Parameters¶
If we want, we could query these functions directly to perform our own parameter scan, but the utility of the design
plugin is that it lets us simply define our design problem as a specification and then takes care of all of the accounting for us.
The first step is to define the design "parameters" (or dimensions), which also serve as inputs to our function defined earlier.
In this case, we have a parameter num
, which is a non-negative integer and a parameter t
, which is a positive float.
We can construct a named tdd.Parameter
for each of these and define some spans as well.
param_num = tdd.ParameterInt(name='num', span=(1, 5))
param_t = tdd.ParameterFloat(name='t', span=(0.1, 0.5), num_points=5)
Note that the name
fields should correspond to the argument names defined in the function.
The float parameter takes an optional num_points
option, which tells the design tool how to discretize the continuous dimension only when doing a grid sweep. For random or Monte Carlo sweeps, it is not used.
Also, it is possible to define parameters that are simply sets of quantities that we might want to select using allowed_values
, such as:
param_str = tdd.ParameterAny(name="some_string", allowed_values=("these", "are", "values"))
but we will ignore this case as it's not needed here and the internal logic is similar to that for integer parameters.
Note: to do more complex sets of integer parameters (like skipping every 'n' integers). We recommend simply using
ParameterAny
and just passing your integers toallowed_values
.
By defining our design parameters like this, we can more concretely define what types and allowed values can be passed to each argument in our function for the parameter sweep.
Method¶
Now that we've defined our parameters, we also need to define the procedure to use to sample the parameter space we've defined. One approach is to independently sample points within the parameter spans, for example using a Monte Carlo method, another is to perform a grid search to uniformly scan between the bounds of each dimension. There are also more complex methods, such as Bayesian optimization and gradient-based optimization, which will be introduced in future releases.
In the design
plugin, we define the specifications for the parameter sweeps using Method
objects.
Here's one example, which defines a grid search.
method = tdd.MethodGrid()
For this example, let's instead do a random (Monte Carlo) sampling with a set number of points.
Note: the default Monte Carlo uses the Latin hypercube sampling method to sample the space. One can also pass an object with a
.random()
method to thesampler
keyword argument ofMethodRandomCustom
for more customization. Other Monte Carlo methods from thescipy
quasi-Monte Carlo engines work well for this and provide more flexibility.
method = tdd.MethodMonteCarlo(num_points=40)
Design Space¶
With the design parameters and our method defined, we can combine everything into a DesignSpace
, which is mainly a container that provides some higher level methods for interacting with these objects.
design_space = tdd.DesignSpace(parameters=[param_num, param_t], method=method)
Now we need to pass our transmission function to the DesignSpace
object to get our results.
To start the parameter scan, the DesignSpace
has two methods .run()
and .run_batch()
, which accept our function(s) defined earlier in the following ways.
-
DesignSpace.run(transmission)
samples the design parameters according to themethod
and then evaluates each point one by one. This is the most general approach becausetransmission
is not restricted to any relationships regardingSimulation
andSimulationData
. Because of this, we can't make any assumptions about whethertransmission
can be parallelized and will need to call it sequentially for each point in the parameter scan. -
On the other hand,
DesignSpace.run_batch(pre, post)
accepts our pre and post processing functions. Because it can assume that one or many simulations are created and run in between, this method can safely construct aweb.Batch
under the hood and run all of the the tasks in parallel over the parameter sweep points. The final results are then stitched together in the end.
Note: the pre processing function can return a single
Simulation
, alist
ortuple
ofSimulation
s or adict
containingSimulation
s. The user just needs to be sure that the post processsing function accepts the correspondingSimulationData
,*args
ofSimulationData
or**kwargs
ofSimulationData
, respectively. For example ifdict(a=sim1, b=sim2)
is returned by the pre-processing function, the post-processing function must have a signature ofdef fn_post(a, b)
.
Results¶
The DesignSpace.run()
and DesignSpace.run_batch()
functions described before both return a Result
object, which is basically a dataset containing the function inputs, outputs, source code, and any task ID information corresponding to each data point.
Note that task ID information can only be gathered when using
run_batch
because in the more generalrun()
function,tidy3d
can't know which tasks were involved in each data point.
results = design_space.run_batch(pre, post)
13:23:37 EST Created task '{'num': 4, 't': 0.4762623720021978}' with task_id 'fdve-7133078e-92cf-4386-baa7-374f28891f74' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-7133078e-92c f-4386-baa7-374f28891f74'.
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:23:38 EST Created task '{'num': 2, 't': 0.4831866916659139}' with task_id 'fdve-ed3a620a-a146-446c-b382-e8af5bd05f8d' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-ed3a620a-a14 6-446c-b382-e8af5bd05f8d'.
13:23:39 EST Created task '{'num': 1, 't': 0.20426746500339904}' with task_id 'fdve-233c6a4d-6c8f-41e2-878f-975146293130' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-233c6a4d-6c8 f-41e2-878f-975146293130'.
13:23:40 EST Created task '{'num': 2, 't': 0.38910786902135}' with task_id 'fdve-e989c016-0698-4992-bd56-b548255bd778' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-e989c016-069 8-4992-bd56-b548255bd778'.
Created task '{'num': 1, 't': 0.22926817565089813}' with task_id 'fdve-a9e41d9b-ff7b-4171-b57e-9b6c3b7f4532' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-a9e41d9b-ff7 b-4171-b57e-9b6c3b7f4532'.
13:23:41 EST Created task '{'num': 3, 't': 0.1508039163318354}' with task_id 'fdve-604c0ac7-cc2c-49f7-b570-3930b6fea7f7' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-604c0ac7-cc2 c-49f7-b570-3930b6fea7f7'.
13:23:42 EST Created task '{'num': 3, 't': 0.34758465812770634}' with task_id 'fdve-5b828505-7697-4fe8-aeb5-c084ade0a371' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-5b828505-769 7-4fe8-aeb5-c084ade0a371'.
Created task '{'num': 4, 't': 0.3110957216164124}' with task_id 'fdve-43ff0410-c1ce-427c-afae-3d3a1b0a57b3' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-43ff0410-c1c e-427c-afae-3d3a1b0a57b3'.
13:23:43 EST Created task '{'num': 4, 't': 0.2105277090223016}' with task_id 'fdve-d268b4ee-f4ba-4063-b3dd-cb0ef059032a' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-d268b4ee-f4b a-4063-b3dd-cb0ef059032a'.
13:23:44 EST Created task '{'num': 4, 't': 0.33943026984843644}' with task_id 'fdve-fce80b0f-b16f-4067-a953-cc0435e5366d' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-fce80b0f-b16 f-4067-a953-cc0435e5366d'.
13:23:45 EST Created task '{'num': 3, 't': 0.49565605894452125}' with task_id 'fdve-df92036d-efee-4d78-8814-af1e9b5380e9' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-df92036d-efe e-4d78-8814-af1e9b5380e9'.
13:23:46 EST Created task '{'num': 3, 't': 0.3534405194096364}' with task_id 'fdve-e8e2f413-07ca-485c-9303-e5f0b53e5f00' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-e8e2f413-07c a-485c-9303-e5f0b53e5f00'.
Created task '{'num': 1, 't': 0.267998780723008}' with task_id 'fdve-988bd226-09a6-46a2-b2d6-3575e1363049' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-988bd226-09a 6-46a2-b2d6-3575e1363049'.
13:23:47 EST Created task '{'num': 1, 't': 0.23442173544508962}' with task_id 'fdve-e22993b8-72ce-41ed-9f99-695825acdabe' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-e22993b8-72c e-41ed-9f99-695825acdabe'.
13:23:48 EST Created task '{'num': 2, 't': 0.11810117128358963}' with task_id 'fdve-6a02d6cf-31b9-453d-9844-84c48510d1a5' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-6a02d6cf-31b 9-453d-9844-84c48510d1a5'.
13:23:49 EST Created task '{'num': 4, 't': 0.2717235106478778}' with task_id 'fdve-a77c35d7-c802-4190-b364-f0a2329a5e49' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-a77c35d7-c80 2-4190-b364-f0a2329a5e49'.
Created task '{'num': 3, 't': 0.12309211309533372}' with task_id 'fdve-7e4fddbf-8b53-4061-998b-26fa64fccc83' and task_type 'FDTD'.
13:23:50 EST View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-7e4fddbf-8b5 3-4061-998b-26fa64fccc83'.
Created task '{'num': 4, 't': 0.17791995937430793}' with task_id 'fdve-9ecb5b16-318c-4bd0-bcb9-4a178a89a261' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-9ecb5b16-318 c-4bd0-bcb9-4a178a89a261'.
13:23:51 EST Created task '{'num': 3, 't': 0.1857928862758346}' with task_id 'fdve-b99f122c-44d4-4d89-9cdd-d7517c74de3d' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-b99f122c-44d 4-4d89-9cdd-d7517c74de3d'.
13:23:52 EST Created task '{'num': 4, 't': 0.2581689943318147}' with task_id 'fdve-719649bb-bc9e-437c-b874-049e982af490' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-719649bb-bc9 e-437c-b874-049e982af490'.
Created task '{'num': 3, 't': 0.4204288586725522}' with task_id 'fdve-028879bc-62dc-474d-b54f-d6e6a93f5bcd' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-028879bc-62d c-474d-b54f-d6e6a93f5bcd'.
13:23:53 EST Created task '{'num': 2, 't': 0.3254282624288959}' with task_id 'fdve-b11d9e1d-5679-4f19-83ac-b837c07712a4' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-b11d9e1d-567 9-4f19-83ac-b837c07712a4'.
13:23:54 EST Created task '{'num': 1, 't': 0.4555145201805667}' with task_id 'fdve-8e21d9e9-14d6-4ae9-ab1c-17ea74f0fc6d' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-8e21d9e9-14d 6-4ae9-ab1c-17ea74f0fc6d'.
Created task '{'num': 4, 't': 0.3770055520897906}' with task_id 'fdve-dc5871b3-0096-4526-9a52-3e744fba1207' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-dc5871b3-009 6-4526-9a52-3e744fba1207'.
13:23:55 EST Created task '{'num': 2, 't': 0.40097468026078953}' with task_id 'fdve-e0ef90b0-134d-4678-b617-7a5cdec61edc' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-e0ef90b0-134 d-4678-b617-7a5cdec61edc'.
13:23:56 EST Created task '{'num': 2, 't': 0.13138177722679795}' with task_id 'fdve-12b7d070-9361-4b42-a5a2-c31937915549' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-12b7d070-936 1-4b42-a5a2-c31937915549'.
13:23:57 EST Created task '{'num': 1, 't': 0.3097651860905113}' with task_id 'fdve-10408fc2-5e70-4b6b-bea2-9f0a601d9d9c' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-10408fc2-5e7 0-4b6b-bea2-9f0a601d9d9c'.
Created task '{'num': 1, 't': 0.4160670223956675}' with task_id 'fdve-17b5b945-d5c1-44df-8e1c-7eaad825896f' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-17b5b945-d5c 1-44df-8e1c-7eaad825896f'.
13:23:58 EST Created task '{'num': 1, 't': 0.44167679929868}' with task_id 'fdve-32df4c42-ed28-45c3-8d93-b8f4176c1d5c' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-32df4c42-ed2 8-45c3-8d93-b8f4176c1d5c'.
13:23:59 EST Created task '{'num': 2, 't': 0.4636438541149702}' with task_id 'fdve-ce5c068f-0cc0-4de3-b62e-d2bbc5b6dcb9' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-ce5c068f-0cc 0-4de3-b62e-d2bbc5b6dcb9'.
13:24:00 EST Created task '{'num': 3, 't': 0.10161088407896583}' with task_id 'fdve-16ac9b97-236f-4c73-ac42-07db918a737c' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-16ac9b97-236 f-4c73-ac42-07db918a737c'.
13:24:01 EST Created task '{'num': 2, 't': 0.2976208591066831}' with task_id 'fdve-86fc1761-1f73-48ce-a4ed-7d5b7440269b' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-86fc1761-1f7 3-48ce-a4ed-7d5b7440269b'.
Created task '{'num': 3, 't': 0.1669886266266093}' with task_id 'fdve-007e51b2-cdb6-4bc2-86b0-1ff99eaf5ab3' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-007e51b2-cdb 6-4bc2-86b0-1ff99eaf5ab3'.
13:24:02 EST Created task '{'num': 1, 't': 0.14646123833398736}' with task_id 'fdve-cf5311da-e64f-4a12-9389-e1fb8018880c' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-cf5311da-e64 f-4a12-9389-e1fb8018880c'.
13:24:03 EST Created task '{'num': 2, 't': 0.3914100599454401}' with task_id 'fdve-a01105f0-67ab-4363-a9e5-a25bf0995efc' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-a01105f0-67a b-4363-a9e5-a25bf0995efc'.
Created task '{'num': 3, 't': 0.24693256427309815}' with task_id 'fdve-4bad075b-8d60-406e-9818-ffa35f9b7c52' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-4bad075b-8d6 0-406e-9818-ffa35f9b7c52'.
13:24:04 EST Created task '{'num': 1, 't': 0.1903124423136173}' with task_id 'fdve-bb17c1fa-0a9e-4174-848d-74f872c836c1' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-bb17c1fa-0a9 e-4174-848d-74f872c836c1'.
13:24:05 EST Created task '{'num': 4, 't': 0.28125275574180264}' with task_id 'fdve-cc209744-f288-42f1-9ccb-dc8cbca80c78' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-cc209744-f28 8-42f1-9ccb-dc8cbca80c78'.
Created task '{'num': 4, 't': 0.3612624461969647}' with task_id 'fdve-02b261eb-b2ba-4e33-8281-f093708089b4' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-02b261eb-b2b a-4e33-8281-f093708089b4'.
13:24:06 EST Created task '{'num': 2, 't': 0.436846395337659}' with task_id 'fdve-ac63bd26-a788-4686-8af8-7f5e7f74109c' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-ac63bd26-a78 8-4686-8af8-7f5e7f74109c'.
13:24:31 EST Started working on Batch.
13:24:50 EST Maximum FlexCredit cost: 1.000 for the whole batch.
Use 'Batch.real_cost()' to get the billed FlexCredit cost after the Batch has completed.
13:25:19 EST Batch complete.
13:25:24 EST loading simulation from ./fdve-7133078e-92cf-4386-baa7-374f28891f74.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:25 EST loading simulation from ./fdve-ed3a620a-a146-446c-b382-e8af5bd05f8d.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:26 EST loading simulation from ./fdve-233c6a4d-6c8f-41e2-878f-975146293130.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:27 EST loading simulation from ./fdve-e989c016-0698-4992-bd56-b548255bd778.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:28 EST loading simulation from ./fdve-a9e41d9b-ff7b-4171-b57e-9b6c3b7f4532.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-604c0ac7-cc2c-49f7-b570-3930b6fea7f7.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:29 EST loading simulation from ./fdve-5b828505-7697-4fe8-aeb5-c084ade0a371.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:30 EST loading simulation from ./fdve-43ff0410-c1ce-427c-afae-3d3a1b0a57b3.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:31 EST loading simulation from ./fdve-d268b4ee-f4ba-4063-b3dd-cb0ef059032a.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:32 EST loading simulation from ./fdve-fce80b0f-b16f-4067-a953-cc0435e5366d.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-df92036d-efee-4d78-8814-af1e9b5380e9.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:33 EST loading simulation from ./fdve-e8e2f413-07ca-485c-9303-e5f0b53e5f00.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:34 EST loading simulation from ./fdve-988bd226-09a6-46a2-b2d6-3575e1363049.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:35 EST loading simulation from ./fdve-e22993b8-72ce-41ed-9f99-695825acdabe.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-6a02d6cf-31b9-453d-9844-84c48510d1a5.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:36 EST loading simulation from ./fdve-a77c35d7-c802-4190-b364-f0a2329a5e49.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:37 EST loading simulation from ./fdve-7e4fddbf-8b53-4061-998b-26fa64fccc83.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:38 EST loading simulation from ./fdve-9ecb5b16-318c-4bd0-bcb9-4a178a89a261.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:39 EST loading simulation from ./fdve-b99f122c-44d4-4d89-9cdd-d7517c74de3d.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:40 EST loading simulation from ./fdve-719649bb-bc9e-437c-b874-049e982af490.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:41 EST loading simulation from ./fdve-028879bc-62dc-474d-b54f-d6e6a93f5bcd.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-b11d9e1d-5679-4f19-83ac-b837c07712a4.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:42 EST loading simulation from ./fdve-8e21d9e9-14d6-4ae9-ab1c-17ea74f0fc6d.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:43 EST loading simulation from ./fdve-dc5871b3-0096-4526-9a52-3e744fba1207.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:44 EST loading simulation from ./fdve-e0ef90b0-134d-4678-b617-7a5cdec61edc.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:45 EST loading simulation from ./fdve-12b7d070-9361-4b42-a5a2-c31937915549.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:46 EST loading simulation from ./fdve-10408fc2-5e70-4b6b-bea2-9f0a601d9d9c.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:47 EST loading simulation from ./fdve-17b5b945-d5c1-44df-8e1c-7eaad825896f.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-32df4c42-ed28-45c3-8d93-b8f4176c1d5c.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:48 EST loading simulation from ./fdve-ce5c068f-0cc0-4de3-b62e-d2bbc5b6dcb9.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:49 EST loading simulation from ./fdve-16ac9b97-236f-4c73-ac42-07db918a737c.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:50 EST loading simulation from ./fdve-86fc1761-1f73-48ce-a4ed-7d5b7440269b.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:51 EST loading simulation from ./fdve-007e51b2-cdb6-4bc2-86b0-1ff99eaf5ab3.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-cf5311da-e64f-4a12-9389-e1fb8018880c.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:52 EST loading simulation from ./fdve-a01105f0-67ab-4363-a9e5-a25bf0995efc.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:53 EST loading simulation from ./fdve-4bad075b-8d60-406e-9818-ffa35f9b7c52.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:54 EST loading simulation from ./fdve-bb17c1fa-0a9e-4174-848d-74f872c836c1.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:55 EST loading simulation from ./fdve-cc209744-f288-42f1-9ccb-dc8cbca80c78.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:56 EST loading simulation from ./fdve-02b261eb-b2ba-4e33-8281-f093708089b4.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:57 EST loading simulation from ./fdve-ac63bd26-a788-4686-8af8-7f5e7f74109c.hdf5
Note: we can pass
verbose=False
to therun_batch()
function to turn off outputs for large batches.
Results
contains three main related datastructures.
-
dims
, which correpsond to thekwargs
of the pre-processing function,('num', 't')
here. -
coords
, which is a tuple containing the values passed in for each of the dims.coords[i]
is a tuple ofn
, andt
values for thei
th function call. -
values
, which is a tuple containing the outputs of the postprocessing functions. In this casevalues[i]
stores the transmission of thei
th function call.
The Results
can be converted to a pandas.DataFrame
where each row is a separate data point and each column is either an input or output for a function. It also contains various methods for plotting and managing the data.
Note: if the measurement function returns a dictionary, the keys will be used as labels for the columns in the
DataFrame
. If it returns atuple
orlist
, each element will get a unique key assigned to them in theDataFrame
depending on the index.
# The first 5 data points
df = results.to_dataframe()
df.head()
num | t | flux | |
---|---|---|---|
0 | 4 | 0.476262 | 0.992865 |
1 | 2 | 0.483187 | 0.999802 |
2 | 1 | 0.204267 | 0.866666 |
3 | 2 | 0.389108 | 0.934000 |
4 | 1 | 0.229268 | 0.862542 |
The pandas
documentation provides excellent explanations of the various postprocessing and visualization functions that can be used.
f, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 3.5))
# plot a hexagonal binning of the results
im = df.plot.hexbin(x="t", y="num", gridsize=10, C="flux", cmap="magma", ax=ax1)
ax1.set_title('SCS (hexagonal binning)')
# scatterplot the raw results with flux on the y axis
im = df.plot.scatter(x="t", y="flux", s=50, c="num", cmap="magma", ax=ax2)
ax2.set_title('SCS (data scatter plot)')
plt.show()
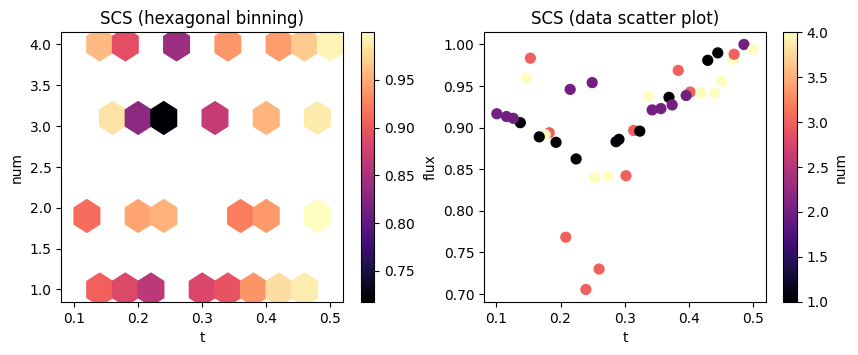
Note, these functions just call pandas.DataFrame
plotting under the hood, so it is worth referring to the docs page on DataFrame visuazation for more tips on plotting design problem results.
Modifying Results¶
After the design problems is run, one might want to modify the results through adding or removing elements or combining different results. Here we will show how to perform some of these advanced features in the design
plugin.
Combining Results¶
We can combine the results of two separate design problems assuming they were created with the same functions.
This can be done either with result.combine(other_result)
or with a shorthand of result + other_result
.
In this example, let's say we want to explore more the region where the transmission seems to be lowest, for t
between 0.2 and 0.3. We create a new parameter with the desired span for t
and use it to create an updated copy of the previous design, still using the same method.
param_t2 = tdd.ParameterFloat(name='t', span=(0.2, 0.3), num_points=5)
design_space2 = design_space.updated_copy(parameters=[param_num, param_t2])
results2 = design_space2.run_batch(pre, post)
Created task '{'num': 3, 't': 0.27233490474804456}' with task_id 'fdve-85a51761-0cbb-4cfb-afe6-bc6b6b7bdb95' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-85a51761-0cb b-4cfb-afe6-bc6b6b7bdb95'.
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:25:58 EST Created task '{'num': 1, 't': 0.23355666061430147}' with task_id 'fdve-806150ff-c2e0-4ce2-a27f-616a69e9d746' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-806150ff-c2e 0-4ce2-a27f-616a69e9d746'.
Created task '{'num': 4, 't': 0.26125339579348794}' with task_id 'fdve-6000c922-798b-4b1f-92dc-26068e14a42a' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-6000c922-798 b-4b1f-92dc-26068e14a42a'.
13:25:59 EST Created task '{'num': 4, 't': 0.2950415554340188}' with task_id 'fdve-8bdbcb1d-146f-4109-b321-1adef7fd3117' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-8bdbcb1d-146 f-4109-b321-1adef7fd3117'.
13:26:00 EST Created task '{'num': 1, 't': 0.28725385837165757}' with task_id 'fdve-9a4e51b1-df81-47cc-8046-6d47766a7cee' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-9a4e51b1-df8 1-47cc-8046-6d47766a7cee'.
13:26:01 EST Created task '{'num': 3, 't': 0.20232105165240377}' with task_id 'fdve-b0738cb6-bb05-4716-8cc0-253dc4233d39' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-b0738cb6-bb0 5-4716-8cc0-253dc4233d39'.
Created task '{'num': 4, 't': 0.22504297504460097}' with task_id 'fdve-4751b461-44fc-446f-8335-3d2558d06403' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-4751b461-44f c-446f-8335-3d2558d06403'.
13:26:02 EST Created task '{'num': 3, 't': 0.27727593683448726}' with task_id 'fdve-90d26982-51c7-469b-b49f-b651666a1ff4' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-90d26982-51c 7-469b-b49f-b651666a1ff4'.
13:26:03 EST Created task '{'num': 4, 't': 0.2519917799524296}' with task_id 'fdve-e01c93f7-82c7-4157-b209-bf3d37c1548a' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-e01c93f7-82c 7-4157-b209-bf3d37c1548a'.
Created task '{'num': 4, 't': 0.2803594829321306}' with task_id 'fdve-eccbc699-2a14-45ae-ad73-b9e3cda08f7c' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-eccbc699-2a1 4-45ae-ad73-b9e3cda08f7c'.
13:26:04 EST Created task '{'num': 3, 't': 0.20296239890283427}' with task_id 'fdve-82c1e140-be5c-4331-9822-1ae9232052c1' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-82c1e140-be5 c-4331-9822-1ae9232052c1'.
13:26:05 EST Created task '{'num': 2, 't': 0.23077214051225334}' with task_id 'fdve-58f2e1df-8497-4fa2-a3a5-5fa7978121a6' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-58f2e1df-849 7-4fa2-a3a5-5fa7978121a6'.
Created task '{'num': 2, 't': 0.29961560051804426}' with task_id 'fdve-c3b2d910-07ff-4036-8a4b-a376598b914f' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-c3b2d910-07f f-4036-8a4b-a376598b914f'.
13:26:06 EST Created task '{'num': 1, 't': 0.22396064654973913}' with task_id 'fdve-5a76b3c0-3086-4c5c-be90-b7753acbc349' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-5a76b3c0-308 6-4c5c-be90-b7753acbc349'.
13:26:07 EST Created task '{'num': 2, 't': 0.26929408931129833}' with task_id 'fdve-f796e077-8364-415c-9cff-e64e3bd2d838' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-f796e077-836 4-415c-9cff-e64e3bd2d838'.
Created task '{'num': 2, 't': 0.23645576705859403}' with task_id 'fdve-69b53292-abca-4ef7-9387-39a871b934f9' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-69b53292-abc a-4ef7-9387-39a871b934f9'.
13:26:08 EST Created task '{'num': 3, 't': 0.2073869949663514}' with task_id 'fdve-259b71be-ae0b-47ec-905b-a990f701d7c3' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-259b71be-ae0 b-47ec-905b-a990f701d7c3'.
13:26:09 EST Created task '{'num': 2, 't': 0.27476170629699764}' with task_id 'fdve-1ec72d16-aed6-4e56-86e5-9f1ce3d593af' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-1ec72d16-aed 6-4e56-86e5-9f1ce3d593af'.
13:26:10 EST Created task '{'num': 2, 't': 0.20943420646257754}' with task_id 'fdve-45975791-54ca-4fef-8406-4d8c0010d6f3' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-45975791-54c a-4fef-8406-4d8c0010d6f3'.
Created task '{'num': 4, 't': 0.21701540944522907}' with task_id 'fdve-2c6d3c8a-3205-43d9-aa30-e1621c566324' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-2c6d3c8a-320 5-43d9-aa30-e1621c566324'.
13:26:11 EST Created task '{'num': 1, 't': 0.2625123679792124}' with task_id 'fdve-38b49381-0826-4d02-856c-910ebdcb9560' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-38b49381-082 6-4d02-856c-910ebdcb9560'.
13:26:12 EST Created task '{'num': 2, 't': 0.2121186970017591}' with task_id 'fdve-e147b819-38ff-4773-b430-0d63960c0cfa' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-e147b819-38f f-4773-b430-0d63960c0cfa'.
13:26:13 EST Created task '{'num': 1, 't': 0.22194060229242474}' with task_id 'fdve-b4824bcc-970f-484a-a586-e684c622cd7a' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-b4824bcc-970 f-484a-a586-e684c622cd7a'.
Created task '{'num': 1, 't': 0.2396742961399555}' with task_id 'fdve-68b08a07-10b3-4df0-a922-5c0b3ec1fa1f' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-68b08a07-10b 3-4df0-a922-5c0b3ec1fa1f'.
13:26:14 EST Created task '{'num': 1, 't': 0.26627688088127743}' with task_id 'fdve-639b8437-c7a6-4032-8a8c-33b69abc6009' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-639b8437-c7a 6-4032-8a8c-33b69abc6009'.
13:26:15 EST Created task '{'num': 3, 't': 0.25729272646706214}' with task_id 'fdve-b2f42658-42ba-4c79-af93-b16fd5cbe1c9' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-b2f42658-42b a-4c79-af93-b16fd5cbe1c9'.
Created task '{'num': 2, 't': 0.21268009937640278}' with task_id 'fdve-5a6c2ff2-c32d-44d9-9873-bc486d1799c2' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-5a6c2ff2-c32 d-44d9-9873-bc486d1799c2'.
13:26:16 EST Created task '{'num': 3, 't': 0.28929583114515406}' with task_id 'fdve-9a48e054-6956-43e3-9620-736809c57440' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-9a48e054-695 6-43e3-9620-736809c57440'.
13:26:17 EST Created task '{'num': 4, 't': 0.29193613258427387}' with task_id 'fdve-0f63dabb-8093-4e7e-a3f0-20e587209d1a' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-0f63dabb-809 3-4e7e-a3f0-20e587209d1a'.
Created task '{'num': 3, 't': 0.2927350156776897}' with task_id 'fdve-7ca387b2-4241-48b4-86e6-f969121eff03' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-7ca387b2-424 1-48b4-86e6-f969121eff03'.
13:26:18 EST Created task '{'num': 1, 't': 0.25332874031852326}' with task_id 'fdve-017505d4-13be-4044-8a33-0bbe27e111df' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-017505d4-13b e-4044-8a33-0bbe27e111df'.
13:26:19 EST Created task '{'num': 4, 't': 0.24544967480289545}' with task_id 'fdve-2b80be55-a076-41e9-b36c-44575f2ff9ce' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-2b80be55-a07 6-41e9-b36c-44575f2ff9ce'.
13:26:20 EST Created task '{'num': 4, 't': 0.2297262672381513}' with task_id 'fdve-52b8ab3a-6ee9-4e4f-9f81-50b8b26ea78f' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-52b8ab3a-6ee 9-4e4f-9f81-50b8b26ea78f'.
Created task '{'num': 3, 't': 0.2413668151484526}' with task_id 'fdve-0c649b99-5b04-4a9f-a00b-d4908683e4bf' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-0c649b99-5b0 4-4a9f-a00b-d4908683e4bf'.
13:26:21 EST Created task '{'num': 2, 't': 0.24943075729606026}' with task_id 'fdve-232d4ffd-7694-4c92-af5d-f2af21d8548e' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-232d4ffd-769 4-4c92-af5d-f2af21d8548e'.
13:26:22 EST Created task '{'num': 3, 't': 0.21901217938980574}' with task_id 'fdve-4c763bc0-aac5-44e8-9306-daf4ed63d92f' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-4c763bc0-aac 5-44e8-9306-daf4ed63d92f'.
Created task '{'num': 2, 't': 0.2435700398935423}' with task_id 'fdve-45158b9e-d6c1-4807-bedc-11e93db3259c' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-45158b9e-d6c 1-4807-bedc-11e93db3259c'.
13:26:23 EST Created task '{'num': 1, 't': 0.27829088286105447}' with task_id 'fdve-c0b0c558-9898-4def-b653-8d83d720a4f4' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-c0b0c558-989 8-4def-b653-8d83d720a4f4'.
13:26:24 EST Created task '{'num': 1, 't': 0.28384071829311586}' with task_id 'fdve-a03d2b06-dfaf-4f12-8567-1d50db94e143' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-a03d2b06-dfa f-4f12-8567-1d50db94e143'.
Created task '{'num': 4, 't': 0.25796100086378376}' with task_id 'fdve-289af330-a112-48e3-876e-113c9d7b38ce' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-289af330-a11 2-48e3-876e-113c9d7b38ce'.
13:26:49 EST Started working on Batch.
13:27:08 EST Maximum FlexCredit cost: 1.000 for the whole batch.
Use 'Batch.real_cost()' to get the billed FlexCredit cost after the Batch has completed.
13:27:36 EST Batch complete.
13:27:41 EST loading simulation from ./fdve-85a51761-0cbb-4cfb-afe6-bc6b6b7bdb95.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:42 EST loading simulation from ./fdve-806150ff-c2e0-4ce2-a27f-616a69e9d746.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:43 EST loading simulation from ./fdve-6000c922-798b-4b1f-92dc-26068e14a42a.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:44 EST loading simulation from ./fdve-8bdbcb1d-146f-4109-b321-1adef7fd3117.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:45 EST loading simulation from ./fdve-9a4e51b1-df81-47cc-8046-6d47766a7cee.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-b0738cb6-bb05-4716-8cc0-253dc4233d39.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:46 EST loading simulation from ./fdve-4751b461-44fc-446f-8335-3d2558d06403.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:47 EST loading simulation from ./fdve-90d26982-51c7-469b-b49f-b651666a1ff4.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:48 EST loading simulation from ./fdve-e01c93f7-82c7-4157-b209-bf3d37c1548a.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:49 EST loading simulation from ./fdve-eccbc699-2a14-45ae-ad73-b9e3cda08f7c.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-82c1e140-be5c-4331-9822-1ae9232052c1.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:50 EST loading simulation from ./fdve-58f2e1df-8497-4fa2-a3a5-5fa7978121a6.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:51 EST loading simulation from ./fdve-c3b2d910-07ff-4036-8a4b-a376598b914f.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:52 EST loading simulation from ./fdve-5a76b3c0-3086-4c5c-be90-b7753acbc349.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-f796e077-8364-415c-9cff-e64e3bd2d838.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:53 EST loading simulation from ./fdve-69b53292-abca-4ef7-9387-39a871b934f9.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:54 EST loading simulation from ./fdve-259b71be-ae0b-47ec-905b-a990f701d7c3.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:55 EST loading simulation from ./fdve-1ec72d16-aed6-4e56-86e5-9f1ce3d593af.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:56 EST loading simulation from ./fdve-45975791-54ca-4fef-8406-4d8c0010d6f3.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-2c6d3c8a-3205-43d9-aa30-e1621c566324.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:57 EST loading simulation from ./fdve-38b49381-0826-4d02-856c-910ebdcb9560.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:58 EST loading simulation from ./fdve-e147b819-38ff-4773-b430-0d63960c0cfa.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:27:59 EST loading simulation from ./fdve-b4824bcc-970f-484a-a586-e684c622cd7a.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-68b08a07-10b3-4df0-a922-5c0b3ec1fa1f.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:00 EST loading simulation from ./fdve-639b8437-c7a6-4032-8a8c-33b69abc6009.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:01 EST loading simulation from ./fdve-b2f42658-42ba-4c79-af93-b16fd5cbe1c9.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:02 EST loading simulation from ./fdve-5a6c2ff2-c32d-44d9-9873-bc486d1799c2.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:03 EST loading simulation from ./fdve-9a48e054-6956-43e3-9620-736809c57440.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-0f63dabb-8093-4e7e-a3f0-20e587209d1a.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:04 EST loading simulation from ./fdve-7ca387b2-4241-48b4-86e6-f969121eff03.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:05 EST loading simulation from ./fdve-017505d4-13be-4044-8a33-0bbe27e111df.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:06 EST loading simulation from ./fdve-2b80be55-a076-41e9-b36c-44575f2ff9ce.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:07 EST loading simulation from ./fdve-52b8ab3a-6ee9-4e4f-9f81-50b8b26ea78f.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-0c649b99-5b04-4a9f-a00b-d4908683e4bf.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:08 EST loading simulation from ./fdve-232d4ffd-7694-4c92-af5d-f2af21d8548e.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:09 EST loading simulation from ./fdve-4c763bc0-aac5-44e8-9306-daf4ed63d92f.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:10 EST loading simulation from ./fdve-45158b9e-d6c1-4807-bedc-11e93db3259c.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
loading simulation from ./fdve-c0b0c558-9898-4def-b653-8d83d720a4f4.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:11 EST loading simulation from ./fdve-a03d2b06-dfaf-4f12-8567-1d50db94e143.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:12 EST loading simulation from ./fdve-289af330-a112-48e3-876e-113c9d7b38ce.hdf5
Now we can inspect the new results and the combined data as we did before:
results_combined = results + results2
df2 = results2.to_dataframe()
df_combined = results_combined.to_dataframe()
f, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 3.5))
im = df2.plot.scatter(x="t", y="flux", s=50, c="num", cmap="magma", ax=ax1)
ax1.set_title('Second run')
im = df_combined.plot.scatter(x="t", y="flux", s=50, c="num", cmap="magma", ax=ax2)
ax1.set_title('Combined results')
plt.show()
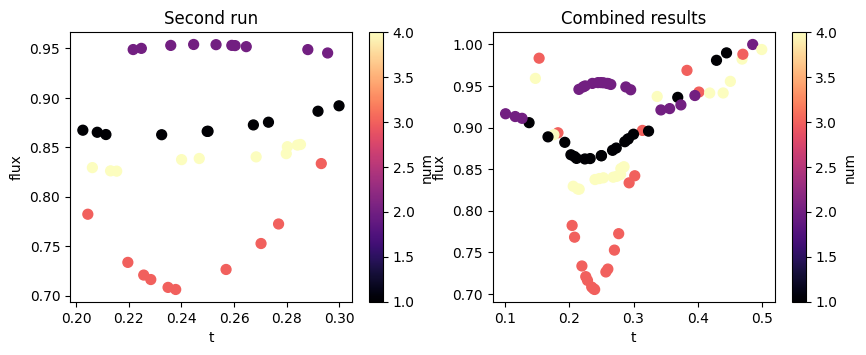
We can also change the method if we want…
# make another set of results using the same function and design but different method
design_space3 = design_space.updated_copy(method=tdd.MethodRandom(num_points=2, monte_carlo_warning=False))
results3 = design_space3.run_batch(pre, post)
Created task '{'num': 2, 't': 0.4421490309736248}' with task_id 'fdve-f7c7cc7f-ac89-4f17-9b51-a2ae1e8b4135' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-f7c7cc7f-ac8 9-4f17-9b51-a2ae1e8b4135'.
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:13 EST Created task '{'num': 2, 't': 0.17280178503859567}' with task_id 'fdve-b93db75d-f2a5-4d53-abc1-9471346ca6e9' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-b93db75d-f2a 5-4d53-abc1-9471346ca6e9'.
13:28:15 EST Started working on Batch.
13:28:16 EST Maximum FlexCredit cost: 0.050 for the whole batch.
Use 'Batch.real_cost()' to get the billed FlexCredit cost after the Batch has completed.
13:28:29 EST Batch complete.
13:28:30 EST loading simulation from ./fdve-f7c7cc7f-ac89-4f17-9b51-a2ae1e8b4135.hdf5
/opt/homebrew/lib/python3.11/site-packages/rich/live.py:229: UserWarning: install "ipywidgets" for Jupyter support warnings.warn('install "ipywidgets" for Jupyter support')
13:28:31 EST loading simulation from ./fdve-b93db75d-f2a5-4d53-abc1-9471346ca6e9.hdf5
… and combine the third run to the previous (now using the combine
method, which is equivalent to adding both):
all_results = results_combined.combine(results3)
print(all_results == results + results2 + results3)
True
Adding and removing results¶
We can also add and remove individual entries from the Results
with the add
and delete
methods.
Let's add a new data point to our results and then delete it.
# define the function inputs and outputs
fn_args = {"t": 1.2, "num": 5}
value = 1.9
To add a data element, we pass in the fn_args
(inputs) and the value
(outputs).
# add it to the results and view the last 5 entries (data is present)
results = results.add(fn_args=fn_args, value=value)
results.to_dataframe().tail()
num | t | flux | |
---|---|---|---|
36 | 1 | 0.190312 | 0.882499 |
37 | 4 | 0.281253 | 0.851173 |
38 | 4 | 0.361262 | 0.995648 |
39 | 2 | 0.436846 | 0.973417 |
40 | 5 | 1.200000 | 1.900000 |
We can select a specific data point by passing the function inputs as keyword arguments to the Results.sel
method.
print(results.sel(**fn_args))
1.9
Similarly, we can remove a data point by passing the fn_args
(inputs) to the Results.delete
method.
# remove this data from the results and view the last 5 entries (data is gone)
results = results.delete(fn_args=fn_args)
results.to_dataframe().tail()
num | t | flux | |
---|---|---|---|
35 | 3 | 0.246933 | 0.717158 |
36 | 1 | 0.190312 | 0.882499 |
37 | 4 | 0.281253 | 0.851173 |
38 | 4 | 0.361262 | 0.995648 |
39 | 2 | 0.436846 | 0.973417 |
Leveraging DataFrame functions¶
Since we have the ability to convert the Results
to and from pandas.DataFrame
objects, we are able to perform various manipulations (including the ones above) on the data using pandas
. For example, we can create a new DataFrame
object that multiplies all of the original flux
values by 1/2 and convert this back to a Result
if desired.
df = results.to_dataframe()
df.head()
num | t | flux | |
---|---|---|---|
0 | 4 | 0.476262 | 0.992865 |
1 | 2 | 0.483187 | 0.999802 |
2 | 1 | 0.204267 | 0.866666 |
3 | 2 | 0.389108 | 0.934000 |
4 | 1 | 0.229268 | 0.862542 |
# get a dataframe where everything is squared
df_half_flux = df.copy()
df_half_flux["flux"] = df_half_flux["flux"].map(lambda x: x * 0.5)
df_half_flux.head()
num | t | flux | |
---|---|---|---|
0 | 4 | 0.476262 | 0.496433 |
1 | 2 | 0.483187 | 0.499901 |
2 | 1 | 0.204267 | 0.433333 |
3 | 2 | 0.389108 | 0.467000 |
4 | 1 | 0.229268 | 0.431271 |
We generally recommend you make use of the many features provided by pandas.DataFrame
to do further postprocessing and analysis of your sweep results, but to convert a DataFrame
back to a Result
, one can simply pass it to Results.from_dataframe
along with the dimensions of the data (if the DataFrame
has been modified).
# load it into a Result, note that we need to pass dims because this is an entirely new DataFrame without metadata
results_squared = tdd.Result.from_dataframe(df_half_flux, dims=("num", "t"))
Accessing the batch data¶
Finally, if Design.run_batch()
was used, then the Result
object also stores the BatchData
associated with the design run.
It can be accessed and iterated through as follows.
batch_data = results.batch_data
batch_data = batch_data.updated_copy(verbose=False)
flux_dict = {}
for task_name, sim_data in batch_data.items():
flux = np.sum(sim_data['flux'].flux.values)
flux_dict[task_name] = flux
from pprint import pprint
pprint(flux_dict)
{"{'num': 1, 't': 0.14646123833398736}": 0.89892215, "{'num': 1, 't': 0.1903124423136173}": 0.8824991, "{'num': 1, 't': 0.20426746500339904}": 0.86666554, "{'num': 1, 't': 0.22926817565089813}": 0.86254245, "{'num': 1, 't': 0.23442173544508962}": 0.86305964, "{'num': 1, 't': 0.267998780723008}": 0.87331647, "{'num': 1, 't': 0.3097651860905113}": 0.88547236, "{'num': 1, 't': 0.4160670223956675}": 0.9721018, "{'num': 1, 't': 0.44167679929868}": 0.9887217, "{'num': 1, 't': 0.4555145201805667}": 0.9948397, "{'num': 2, 't': 0.11810117128358963}": 0.9126044, "{'num': 2, 't': 0.13138177722679795}": 0.90948576, "{'num': 2, 't': 0.2976208591066831}": 0.9443503, "{'num': 2, 't': 0.3254282624288959}": 0.92364144, "{'num': 2, 't': 0.38910786902135}": 0.93400043, "{'num': 2, 't': 0.3914100599454401}": 0.9358025, "{'num': 2, 't': 0.40097468026078953}": 0.93747365, "{'num': 2, 't': 0.436846395337659}": 0.9734174, "{'num': 2, 't': 0.4636438541149702}": 0.99401253, "{'num': 2, 't': 0.4831866916659139}": 0.9998017, "{'num': 3, 't': 0.10161088407896583}": 0.9450594, "{'num': 3, 't': 0.12309211309533372}": 0.98991394, "{'num': 3, 't': 0.1508039163318354}": 0.9873391, "{'num': 3, 't': 0.1669886266266093}": 0.9499912, "{'num': 3, 't': 0.1857928862758346}": 0.8792661, "{'num': 3, 't': 0.24693256427309815}": 0.7171576, "{'num': 3, 't': 0.34758465812770634}": 0.9968836, "{'num': 3, 't': 0.3534405194096364}": 0.999848, "{'num': 3, 't': 0.4204288586725522}": 0.9341517, "{'num': 3, 't': 0.49565605894452125}": 0.9973784, "{'num': 4, 't': 0.17791995937430793}": 0.8869701, "{'num': 4, 't': 0.2105277090223016}": 0.8273418, "{'num': 4, 't': 0.2581689943318147}": 0.84047914, "{'num': 4, 't': 0.2717235106478778}": 0.84109175, "{'num': 4, 't': 0.28125275574180264}": 0.8511734, "{'num': 4, 't': 0.3110957216164124}": 0.8654079, "{'num': 4, 't': 0.33943026984843644}": 0.94703233, "{'num': 4, 't': 0.3612624461969647}": 0.99564797, "{'num': 4, 't': 0.3770055520897906}": 0.9967587, "{'num': 4, 't': 0.4762623720021978}": 0.9928651}